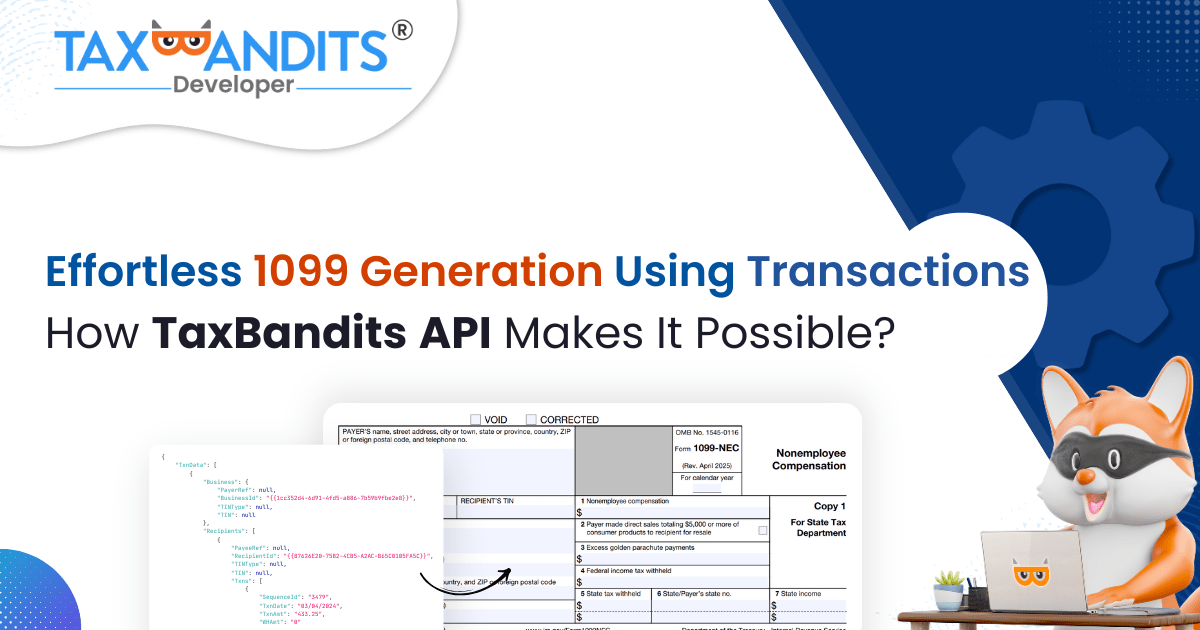
Effortless 1099 Generation Using Transactions: How TaxBandits API Makes It Possible
Tax season can be stressful for many businesses, particularly when it comes to filing 1099 forms. The pressure to ensure timely, accurate filings while managing an increasing volume of contractor payments is no small task.